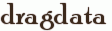

A dragdata object contains the information transferred when a drag and drop operation is performed between two
user interface elements.
The type property must be defined and determines how the receiving element will process the information. The types supported by the
framework are "number", "string", "dice", "shortcut" and "token", all containing (only) the respective data. Custom types can be identified by
any string, but the use of as unambiguous identifiers as possible, considering the purpose, is recommended.
The actual data consists of one or more slots, and each slot contains a combination of the available data items (number,
string, shortcut, token and database node). All built in types support only the first slot. It is up to the scripts handling custom
types to interpret the data contained in the slots correctly.
Each instance of this object also optionally contains a description string and an icon resource name. The description can be used
by elements such as the chat window and hot key bar to apply a descriptive label to the entirety of the drag data. The icon can be used
to set a bitmap to be drawn at the mouse cursor while the drag is underway.
If the drag contains dice, all of them are drawn at the cursor. If there are no dice and an icon has been specified, the icon graphic
will be used. In the absence of the above, the token prototype graphic is used if a token is defined. If none of the latter information
is available, the string value, or finally, the number value, will be used for representing the drag.
To support specialized operations in cases where a default function is applicable, a base dragdata inherited object can
be created. An example of such a case could be a token control that attaches extra information to a token, but it is still desired that
the drag can be used as a framework supported "token" drag if the custom type is not supported by the target element. To implement this,
the custom data should first be set, and the createBaseData function used to create the base object. The returned reference
to the base object can then be used to set up data matching a built in type. Base objects can be chained.
The function isType should generally be used by all elements to check the type of a drop operation. It automatically causes
further data reads from the object to return information from a matching base object. This functionality is also utilized by the framework,
i.e. a custom drag with a supported base data item available in the chain can be used with an out of the box framework element. The
currently active data is called top level data. Special cases requiring a rewind of the inheritance chain can use
the resetType function.
When drag objects are set using the setData function, here is the expected format of the LUA table used to define the drag object:
-
type: String. Drag object type.
-
icon: String. Icon associated with this drag object.
-
description: String. Description associated with this drag object.
-
dbref: String (or databasenode). Data base node identifier (or databasenode object) associated with this drag object.
-
secret: Boolean. Flag indicating whether drag data should be displayed local only when dropped on chat window.
-
shortcuts: Table of shortcut records. Each shortcut record is a table containing class and recordname string fields. Any shortcut records associated with this drag object.
-
slots: Table of slot records. See below.
Each slot record is a LUA table with the following fields:
-
type: String. Slot type.
-
number: Number. Numeric value associated with this slot.
-
string: String. Textual value associated with this slot.
-
token: String. Token identified associated with this slot.
-
shortcut: Table. Shortcut associated with this slot. Each shortcut record is a table containing class and recordname string fields.
-
dice: Table. Dice associated with this slot. The table contains numerically indexed die resource names.
-
metadata: Table. Metadata associated with this slot.
-
custom: Object. Custom LUA variable associated with this slot. This information will not be saved for drag objects placed on hot key bar.
Interface
addDie
function addDie(die)
Adds a single die value to the currently active slot. The existing dice in the slot will be preserved, and the new die appended to the slot die list.
Parameters
die (string)
A string indicating the die asset to add
addShortcut
function addShortcut(class, record)
Adds a shortcut record to the list of shortcut records for this drag object.
Parameters
class (string)
Windowclass to use when opening the shortcut link
record (string)
Data base node identifier to use when opening the shortcut link
createBaseData
function createBaseData([type])
Create a new dragdata object as an inherited base data to the current top level data.
Existing base data is destroyed.
Parameters
type (string) [optional]
The type applied to the created base dragdata
Return values
(dragdata)
Returns a
dragdata object representing the created base data object.
disableHotkeying
function disableHotkeying(state)
This function can be used to indicate that the current dragdata can not be dropped in the hot key bar. This is useful
if the drag contains custom data, or other references that might not be valid across several sessions.
Parameters
state (boolean)
A true value to indicate that hotkeying should be disabled, or false to
enable hotkeying
getCustomData
function getCustomData()
Retrieves the Lua variable stored in the custom data value in the currently active slot in the top level data.
Return values
(any)
A variable stored in the active slot or nil if no custom variable has been set
getDatabaseNode
function getDatabaseNode()
Get the database node associated with the dragdata object.
The database node returned will either be last value set by setDatabaseNode or setShortcutData (even though setShortcutData is set by slot).
Return values
(databasenode)
getDescription
function getDescription()
Retrieve the description for the entire drag item.
Return values
(string)
The description string
getDieList
function getDieList()
Get the list of dice in the currently active slot in the top level data. The result is an integer indexed table
of table values specifying the data related to the dice. The subtable will contain the field type
that is a string identifying the type of die, and a field named result containing the numeric
result of the die roll if the dice have already been rolled.
Return values
(table)
An integer indexed table of data containing the die types and possibly results
getMetaData
function getMetaData(key)
Returns the requested meta data attribute from the current slot.
Parameters
key (string)
Meta data key
Return values
(string)
Meta data value stored under the specified key
getMetaDataList
function getMetaDataList()
Returns a table of meta data attributes for the current slot.
Return values
(table)
Table of meta data attributes, where the each key is the attribute name, and each value is the attribute value string for that key
getNumberData
function getNumberData()
Retrieves the number value in the currently active slot in the top level data.
Return values
(number)
Returns the number value
getSecret
function getSecret()
Returns whether the secret flag is set on the drag object. By default, objects dragged onto the chat window will only show locally if this flag set.
Return values
(string)
Returns true if the secret flag is set for this drag object; otherwise, returns false.
getShortcutData
function getShortcutData()
Retrieves the shortcut value in the currently active slot in the top level data.
Return values
(string)
The windowclass for the shortcut data or nil if no shortcut has been specified
(string)
The database node identifier for the shortcut target or nil if no shortcut
has been specified
getShortcutList
function getShortcutList()
Returns the list of shortcut records stored for this drag object.
Return values
(table)
Table of shortcut records, where each record is a table with 2 values stored under class and recordname keys.
getSlot
function getSlot()
Get the currently active slot's index number.
Return values
(number)
The current index slot in the range 1 .. (number of slots)
getSlotCount
function getSlotCount()
Get the number of slots in the dragdata object
Return values
(number)
The number of slots
getSlotType
function getSlotType()
Returns the type attribute of the current slot.
Return values
(string)
The string specifying the slot type
getStringData
function getStringData()
Retrieves the string value in the currently active slot in the top level data.
Return values
(string)
Returns the string value
getTokenData
function getTokenData()
Retrieves the value of the token prototype identifier string in the currently active slot in the top level data.
Return values
(any)
The string identifying the token prototype, or nil if no token is contained in the data
getType
function getType()
Returns the type string of the current top level data without performing checks on the inheritance chain.
Return values
(string)
The string specifying the data type
isType
function isType(type)
Check the inheritance chain for matching types to the type given as a parameter, starting at the current
top level data. If a match is found, the match is set as the current top level data.
Parameters
type (string)
The type being sought
Return values
(boolean)
If a match is found, returns true, otherwise returns false.
nextSlot
function nextSlot()
Increments the slot counter by one, if there are more slots available.
Return values
(boolean)
Returns true if successful, or false if the operation fails because
the current slot is the last one
reset
function reset()
Delete and reset all properties of the drag data item. The type field must be set after this operation for the
object to represent valid drag contents.
resetType
function resetType()
Set the highest level data as the current top level element.
revealDice
function revealDice(state)
This function can be used to indicate that GM rolls, which are by default hidden, should be displayed directly to clients.
This function has no effect if the drag does not contain dice or does not cause a roll of the dice.
Parameters
state (boolean)
A value of true to reveal the dice, or false to make a hidden roll
setCustomData
function setCustomData(variable)
Sets an arbitrary Lua variable into the custom data value in the currently active slot in the top level data.
Parameters
variable (any)
Any variable
setData
function setData(dragrecord)
Set the drag object to the attributes specified in the drag record.
Parameters
dragrecord (table)
See dragdata description for details on the drag record structure.
setDatabaseNode
function setDatabaseNode(nodename)
Set the a data node identifier path to be associated with the dragdata object.
Parameters
nodename (string)
A data mode identifier
setDatabaseNode
function setDatabaseNode(node)
Set a database node to be associated with the dragdata object.
Parameters
node (databasenode)
A databasenode object
setDescription
function setDescription(description)
Set the string used as a label for the entire drag item. This data is shared between all slots in the object.
Parameters
description (string)
The description string
setDieList
function setDieList(dielist)
Set the list of dice in the currently active slot in the top level data. The existing dice in the slot will be
removed and the set of held dice in all slots will be rebuilt.
Parameters
dielist (table)
An integer indexed table of strings listing the types of the dice to be added to the slot.
setIcon
function setIcon(icon)
Set the name of the icon resource used to render a graphic at the mouse cursor while the drag is taking place.
Parameters
icon (string)
The name of an icon resource used for the icon
setMetaData
function setMetaData(key, value)
Sets the given meta data attribute in the current slot.
Parameters
key (string)
Meta data key
value (string)
Meta data value
setNumberData
function setNumberData(value)
Sets the number value in the currently active slot in the top level data.
Parameters
value (number)
The desired number value
setSecret
function setSecret(value)
Specifies whether the secret flag is set on the drag object. By default, objects dragged onto the chat window will only show locally if this flag set.
Parameters
value (boolean)
Set to true to mark the drag data as secret; otherwise, set to false.
setShortcutData
function setShortcutData(class, recordname)
Sets the shortcut value in the currently active slot in the top level data. The value consists of a windowclass
name and an absolute database node identifier.
Parameters
class (string)
The name of the windowclass resource used as a target of the shortcut
recordname (string)
The database node identifier used to construct the target data source
setSlot
function setSlot(index)
Set the slot counter to the specified index. The index can be any positive integer, if it is smaller than the largest slot
index, the number of slots is adjusted to match the given index.
Parameters
index (number)
The new slot index
Return values
(boolean)
Returns true if successful, or false if the operation fails because
the specified slot index is less than 1
setSlotType
function setSlotType(type)
Sets the type attribute of the current slot.
Parameters
type (string)
The new value used as the type of the current slot
setStringData
function setStringData(value)
Sets the string value in the currently active slot in the top level data.
Parameters
value (string)
The desired string value
setTokenData
function setTokenData(prototypename)
Sets the token prototype identifier string value in the currently active slot in the top level data. Only strings
obtained through secondary token sources (such as other tokencontrol instances or token containers) should be used as
the parameter.
Parameters
prototypename (string)
The string identifying the token prototype to add to the data
setType
function setType(type)
Set the type string.
Parameters
type (string)
The new value used as the type of the object